スクリーンショットを撮る簡単なコンソールC#プログラム
コンソールからデスクトップのスクリーショットを撮って表示するC#プログラムです。Windows専用なのでプロジェクトのターゲットOSをWindows7以上に設定してください。
Nuget情報
Llavaの時と同じくコンソールに画像表示するためにSpectre.Consoleを使います。
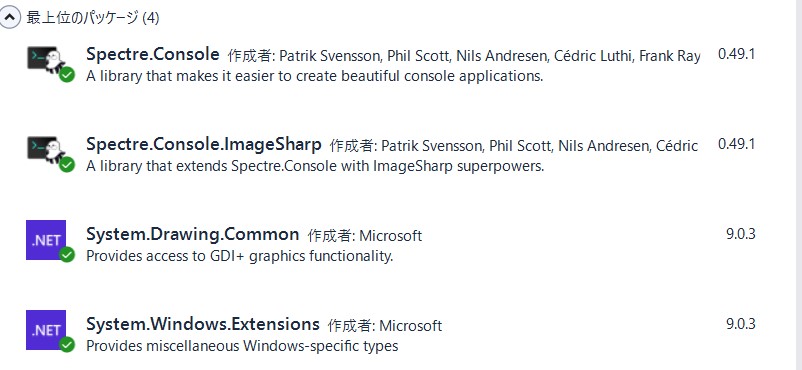
Program.cs
デスクトップのスクリーショットを撮ってコンソールに表示します。
using System.Drawing;
using System.Drawing.Imaging;
using System.Runtime.InteropServices;
using Spectre.Console;
namespace ScreenTest
{
class Program
{
static void Main(string[] args)
{
while (true)
{
Image img = CaptureScreen();
Bitmap bitmap = new Bitmap(img);
MemoryStream ms = new MemoryStream();
bitmap.Save(ms, ImageFormat.Png);
CanvasImage consoleImage = new CanvasImage(ms.ToArray());
consoleImage.MaxWidth = 50;
AnsiConsole.Write(consoleImage);
ms.Dispose();
Console.Write("Input:");
string strInput = Console.ReadLine() ?? "";
if (strInput == "exit") break; // 'exit'と入力したら終わり
}
}
private static Image CaptureScreen()
{
return CaptureWindow(GetDesktopWindow());
}
private static Image CaptureWindow(IntPtr handle)
{
IntPtr hdcSrc = GetWindowDC(handle);
RECT windowRect = new RECT();
GetWindowRect(handle, ref windowRect);
int width = windowRect.right - windowRect.left;
int height = windowRect.bottom - windowRect.top;
IntPtr hdcDest = CreateCompatibleDC(hdcSrc);
IntPtr hBitmap = CreateCompatibleBitmap(hdcSrc, width, height);
IntPtr hOld = SelectObject(hdcDest, hBitmap);
BitBlt(hdcDest, 0, 0, width, height, hdcSrc, 0, 0, SRCCOPY);
SelectObject(hdcDest, hOld);
DeleteDC(hdcDest);
ReleaseDC(handle, hdcSrc);
Image img = Image.FromHbitmap(hBitmap);
DeleteObject(hBitmap);
return img;
}
[StructLayout(LayoutKind.Sequential)]
private struct RECT
{
public int left;
public int top;
public int right;
public int bottom;
}
private const int SRCCOPY = 0x00CC0020; // BitBlt dwRop parameter
[DllImport("gdi32.dll")]
private static extern bool BitBlt(IntPtr hObject, int nXDest, int nYDest,
int nWidth, int nHeight, IntPtr hObjectSource,
int nXSrc, int nYSrc, int dwRop);
[DllImport("gdi32.dll")]
private static extern IntPtr CreateCompatibleBitmap(IntPtr hDC, int nWidth,
int nHeight);
[DllImport("gdi32.dll")]
private static extern IntPtr CreateCompatibleDC(IntPtr hDC);
[DllImport("gdi32.dll")]
private static extern bool DeleteDC(IntPtr hDC);
[DllImport("gdi32.dll")]
private static extern bool DeleteObject(IntPtr hObject);
[DllImport("gdi32.dll")]
private static extern IntPtr SelectObject(IntPtr hDC, IntPtr hObject);
[DllImport("user32.dll")]
private static extern IntPtr GetDesktopWindow();
[DllImport("user32.dll")]
private static extern IntPtr GetWindowDC(IntPtr hWnd);
[DllImport("user32.dll")]
private static extern IntPtr ReleaseDC(IntPtr hWnd, IntPtr hDC);
[DllImport("user32.dll")]
private static extern IntPtr GetWindowRect(IntPtr hWnd, ref RECT rect);
}
}
実行結果
犬の写真をデスクトップに表示させてスクリーショットを撮りました。コンソール表示は荒いですが内部ではちゃんと鮮明に撮れています。
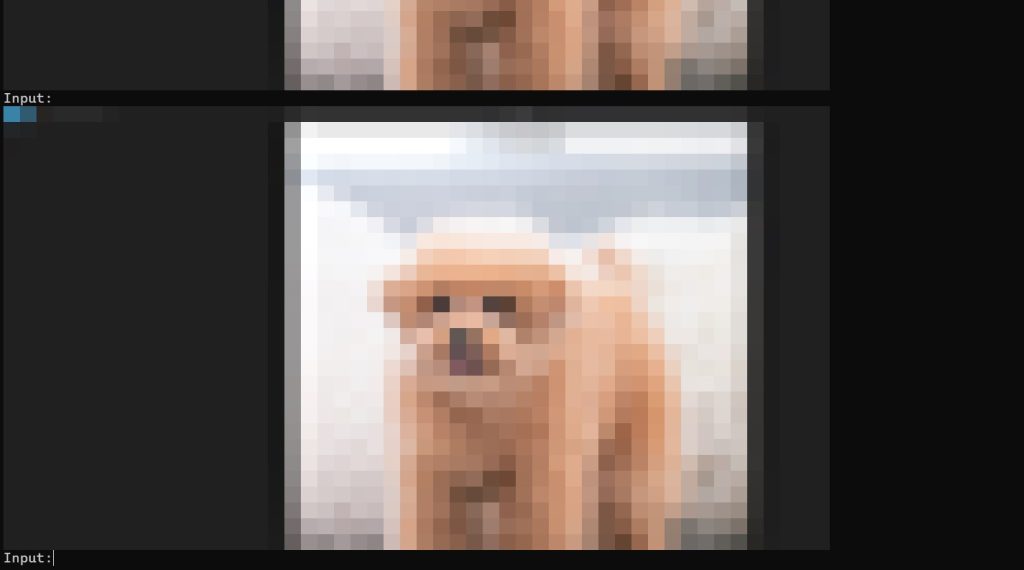